Docker Containers#
Readings: [Docker Guide]
Introduction#
Containerization solves the problem of running applications consistently with multiple dependencies on the same machine, or across multiple machines, by enabling reproducible builds of applications running in lightweight isolated environments. Moreover, containers can be easily pulled by other machines from a container registry. This is important for development and collaboration. Note that this assumes each machine runs a container runtime. In this notebook, we will use Docker which provides an ecosystem for efficiently working with containers.
Hello world#
The following example demonstrates building and running a container:
!docker run hello-world
Unable to find image 'hello-world:latest' locally
latest: Pulling from library/hello-world
fc919002: Pull complete 195kB/3.195kBBDigest: sha256:ac69084025c660510933cca701f615283cdbb3aa0963188770b54c31c8962493
Status: Downloaded newer image for hello-world:latest
Hello from Docker!
This message shows that your installation appears to be working correctly.
To generate this message, Docker took the following steps:
1. The Docker client contacted the Docker daemon.
2. The Docker daemon pulled the "hello-world" image from the Docker Hub.
(arm64v8)
3. The Docker daemon created a new container from that image which runs the
executable that produces the output you are currently reading.
4. The Docker daemon streamed that output to the Docker client, which sent it
to your terminal.
To try something more ambitious, you can run an Ubuntu container with:
$ docker run -it ubuntu bash
Share images, automate workflows, and more with a free Docker ID:
https://hub.docker.com/
For more examples and ideas, visit:
https://docs.docker.com/get-started/
The above message tells the entire process of how the hello-world
container eventually is able to run on our machine. The image was pulled on Docker Hub which is a registry of Docker images. Note that the creation of images occurs locally since the local machine is also our compute layer.
The container proceeds to run its default command, i.e. execute the /hello
program that prints the message on the terminal. The hello-world
image produces a minimal container whose sole purpose is to print this message.
Fig. 125 Anatomy of a Docker image and the resulting hello-world
container in the context of the Linux kernel. Note the specific partition on the hard disk for the filesystem of the image.#
An image is essentially a filesystem snapshot with startup commands. This can be thought of as a read-only template which provides the daemon a set of instructions for creating a container. A container on the other hand is a running process in the Linux VM with partitioned hardware resources allocated by the kernel.
Remark. It would be significantly faster to run the hello-world
container a second time since Docker uses a cache to build it. This makes sense since multiple containers are usually created from the same image.
Interactive mode#
As mentioned, containers have isolated filesystems by default. This means we can blow up a container and just create a fresh healthy container from the same image. This also ensures that our running processes will not affect the host computer which can be running other important processes. Running an ubuntu container in detached (-d
) and interactive mode (-it
):
!docker run -d -it --name ubuntu0 ubuntu
Unable to find image 'ubuntu:latest' locally
latest: Pulling from library/ubuntu
Digest: sha256:6042500cf4b44023ea1894effe7890666b0c5c7871ed83a97c36c76ae560bb9b[1A
Status: Downloaded newer image for ubuntu:latest
01aa51ea6dee2d6979609d9f3695ed0a150723283943c64c41a40e86867acaab
This allows us to use the CLI inside the container using docker exec
:
!docker exec ubuntu0 ls -C
!docker exec ubuntu0 rm -rf bin/ls
!docker exec ubuntu0 ls -C
!docker stop ubuntu0 > /dev/null
bin dev home media opt root sbin sys usr
boot etc lib mnt proc run srv tmp var
OCI runtime exec failed: exec failed: unable to start container process: exec: "ls": executable file not found in $PATH: unknown
Creating a fresh container that can run ls
. Note that the container ID is different:
!docker run -d -it --name ubuntu1 ubuntu
!docker exec ubuntu1 ls -C
!docker stop ubuntu1 > /dev/null
68379450fe0cd1e6ae70bd30b739107f8e0d235bc7a0c0325498cd3ce3e12537
bin dev home media opt root sbin sys usr
boot etc lib mnt proc run srv tmp var
Other commands#
Listing all containers and images:
!docker ps --all
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
68379450fe0c ubuntu "/bin/bash" 12 seconds ago Exited (137) Less than a second ago ubuntu1
01aa51ea6dee ubuntu "/bin/bash" 25 seconds ago Exited (137) 12 seconds ago ubuntu0
587138c99675 hello-world "/hello" 42 seconds ago Exited (0) 41 seconds ago confident_gagarin
!docker image ls
REPOSITORY TAG IMAGE ID CREATED SIZE
ubuntu latest da935f064913 2 weeks ago 69.3MB
hello-world latest ee301c921b8a 7 months ago 9.14kB
Remark. The hello-world
container immediately exited after running with exit status zero since no errors were encountered. The other containers continue running since they are run in interactive mode.
To stop containers, we can use either stop
or kill
. The stop
command sends a SIGTERM to the running process. This gives 10 seconds for cleanup, then a fallback SIGKILL is sent to immediately terminate the process. See Fig. 126 and restart policies docs.
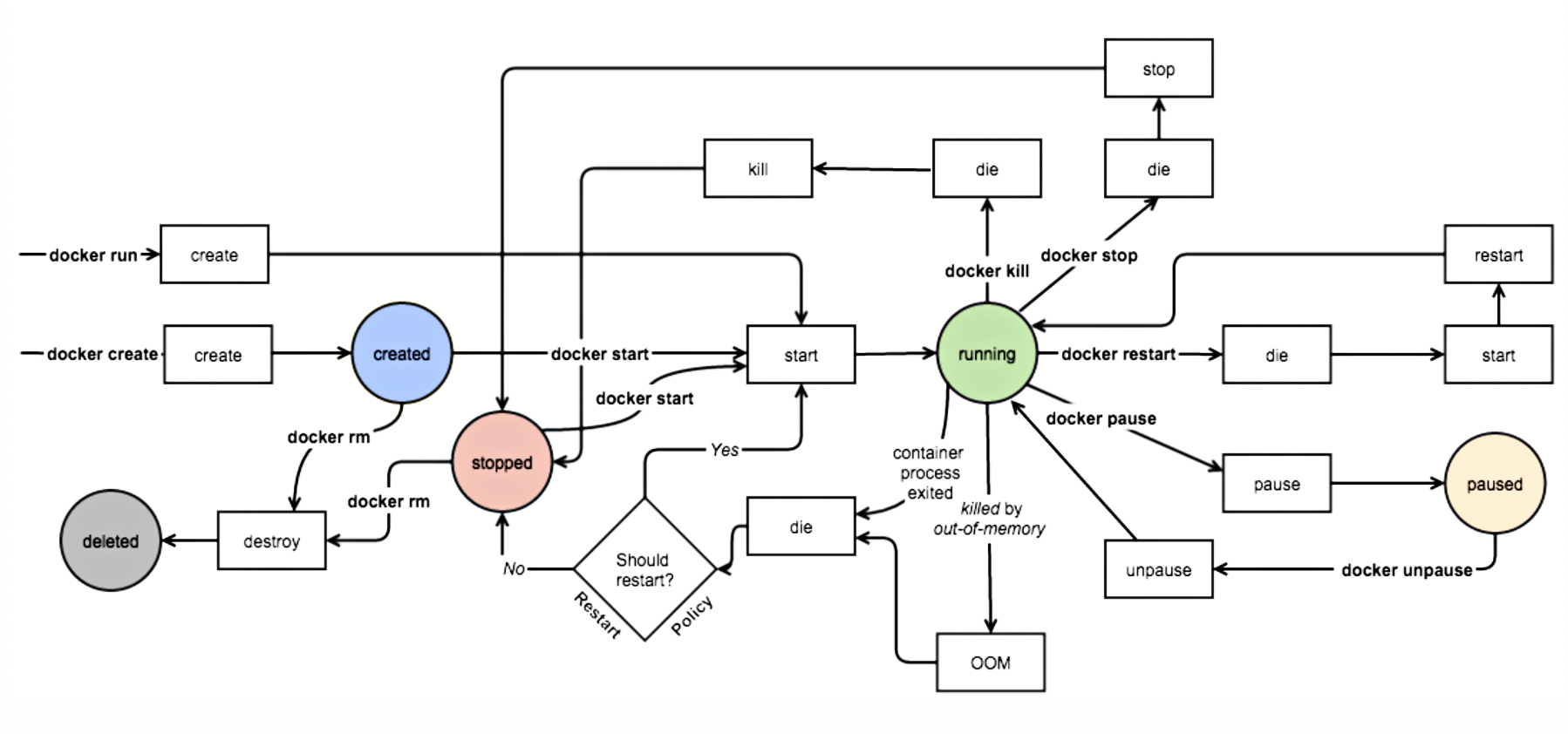
Docker build#
Throughout the above examples we have been using public images from Docker Hub.
In this section, we create our own images for running our own containers. Our custom images can be pushed to container
repositories, such as Docker Hub or ECR, which our servers can pull
to run our containers remotely. To do this, Docker requires us to create a Dockerfile
which specifies the container build process.
import os; os.chdir("./containers/")
!tree ./simple-fastapi -I __pycache__
./simple-fastapi
├── Dockerfile
├── requirements.txt
└── src
└── main.py
2 directories, 3 files
The web app simply prints a message when the root URI is called:
!pygmentize ./simple-fastapi/src/main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def root():
return {"message": "Hello world!"}
Dockerfile#
The following Dockerfile
uses python:3.10-slim
as base image. This slim
image is a smaller version of a container running Python 3.10, but still larger than alpine
. The next lines serve to modify the base image. First, it specifies /code
as the working directory. This is where all subsequent build commands will be executed.
The copy command copies files in the build folder to a path relative to the working directory. Next, we call pip
with certain flags so that it does not cache the installs, making the container smaller. Note that we use ENTRYPOINT
instead of CMD
. The latter can be overridden during run.
!pygmentize ./simple-fastapi/Dockerfile
FROM python:3.10-slim
WORKDIR /code
COPY ./requirements.txt ./
RUN pip install --no-cache-dir --upgrade -r requirements.txt
COPY ./src ./src
ENTRYPOINT ["uvicorn", "src.main:app", "--host", "0.0.0.0", "--port", "80"]
Building the image. Using the -t
flag, we add an image path (this defaults to the latest
tag):
!docker build ./simple-fastapi -t okt/simple-fastapi
Show code cell output
?25l[+] Building 0.0s (0/1)
?25h?25l[+] Building 0.1s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.0s
?25h?25l[+] Building 0.3s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.2s
?25h?25l[+] Building 0.4s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.3s
?25h?25l[+] Building 0.6s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.5s
?25h?25l[+] Building 0.7s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.6s
?25h?25l[+] Building 0.9s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.8s
?25h?25l[+] Building 1.0s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.9s
?25h?25l[+] Building 1.2s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.1s
?25h?25l[+] Building 1.3s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.2s
?25h?25l[+] Building 1.5s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.4s
?25h?25l[+] Building 1.6s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.5s
?25h?25l[+] Building 1.8s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.7s
?25h?25l[+] Building 1.9s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.8s
?25h?25l[+] Building 2.1s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 2.0s
?25h?25l[+] Building 2.2s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 2.1s
?25h?25l[+] Building 2.4s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 2.3s
?25h?25l[+] Building 2.5s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 2.4s
?25h?25l[+] Building 2.6s (5/9)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 2.5s
=> [internal] load build context 0.0s
=> => transferring context: 627B 0.0s
=> [1/5] FROM docker.io/library/python:3.10-slim@sha256:25f03d17398b3f00 0.0s
?25h?25l[+] Building 2.7s (10/10) FINISHED
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 37B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 2.5s
=> [internal] load build context 0.0s
=> => transferring context: 627B 0.0s
=> [1/5] FROM docker.io/library/python:3.10-slim@sha256:25f03d17398b3f00 0.0s
=> CACHED [2/5] WORKDIR /code 0.0s
=> CACHED [3/5] COPY ./requirements.txt ./ 0.0s
=> CACHED [4/5] RUN pip install --no-cache-dir --upgrade -r requirements 0.0s
=> CACHED [5/5] COPY ./src ./src 0.0s
=> exporting to image 0.0s
=> => exporting layers 0.0s
=> => writing image sha256:67acde1baf9495ebb79bccb14f5c34f6b114958bfab1c 0.0s
=> => naming to docker.io/okt/simple-fastapi 0.0s
?25h
Cached layers#
The order of Dockerfile instructions matters. Each instruction in a Dockerfile roughly translates to an image layer. The layers are cached in the build process. Hence, changes in one layer destroys the cache of subsequent layers. This is known as cache busting. This explains why requirements are installed first in the Dockerfile
before source code is copied, so that modifying the source does not result in reinstalling the dependencies (Fig. 128).
The following created timestamps indicate cached layers in our previous build:
!docker history okt/simple-fastapi
IMAGE CREATED CREATED BY SIZE COMMENT
67acde1baf94 About an hour ago ENTRYPOINT ["uvicorn" "src.main:app" "--host… 0B buildkit.dockerfile.v0
<missing> About an hour ago COPY ./src ./src # buildkit 425B buildkit.dockerfile.v0
<missing> 4 hours ago RUN /bin/sh -c pip install --no-cache-dir --… 18.7MB buildkit.dockerfile.v0
<missing> 4 hours ago COPY ./requirements.txt ./ # buildkit 25B buildkit.dockerfile.v0
<missing> 4 hours ago WORKDIR /code 0B buildkit.dockerfile.v0
<missing> 2 months ago CMD ["python3"] 0B buildkit.dockerfile.v0
<missing> 2 months ago RUN /bin/sh -c set -eux; savedAptMark="$(a… 12.2MB buildkit.dockerfile.v0
<missing> 2 months ago ENV PYTHON_GET_PIP_SHA256=9cc01665956d22b3bf… 0B buildkit.dockerfile.v0
<missing> 2 months ago ENV PYTHON_GET_PIP_URL=https://github.com/py… 0B buildkit.dockerfile.v0
<missing> 2 months ago ENV PYTHON_SETUPTOOLS_VERSION=65.5.1 0B buildkit.dockerfile.v0
<missing> 2 months ago ENV PYTHON_PIP_VERSION=23.0.1 0B buildkit.dockerfile.v0
<missing> 2 months ago RUN /bin/sh -c set -eux; for src in idle3 p… 32B buildkit.dockerfile.v0
<missing> 2 months ago RUN /bin/sh -c set -eux; savedAptMark="$(a… 35.5MB buildkit.dockerfile.v0
<missing> 2 months ago ENV PYTHON_VERSION=3.10.13 0B buildkit.dockerfile.v0
<missing> 2 months ago ENV GPG_KEY=A035C8C19219BA821ECEA86B64E628F8… 0B buildkit.dockerfile.v0
<missing> 2 months ago RUN /bin/sh -c set -eux; apt-get update; a… 9.11MB buildkit.dockerfile.v0
<missing> 2 months ago ENV LANG=C.UTF-8 0B buildkit.dockerfile.v0
<missing> 2 months ago ENV PATH=/usr/local/bin:/usr/local/sbin:/usr… 0B buildkit.dockerfile.v0
<missing> 8 days ago /bin/sh -c #(nop) CMD ["bash"] 0B
<missing> 8 days ago /bin/sh -c #(nop) ADD file:262fd7bf0bc26e5d2… 97.1MB
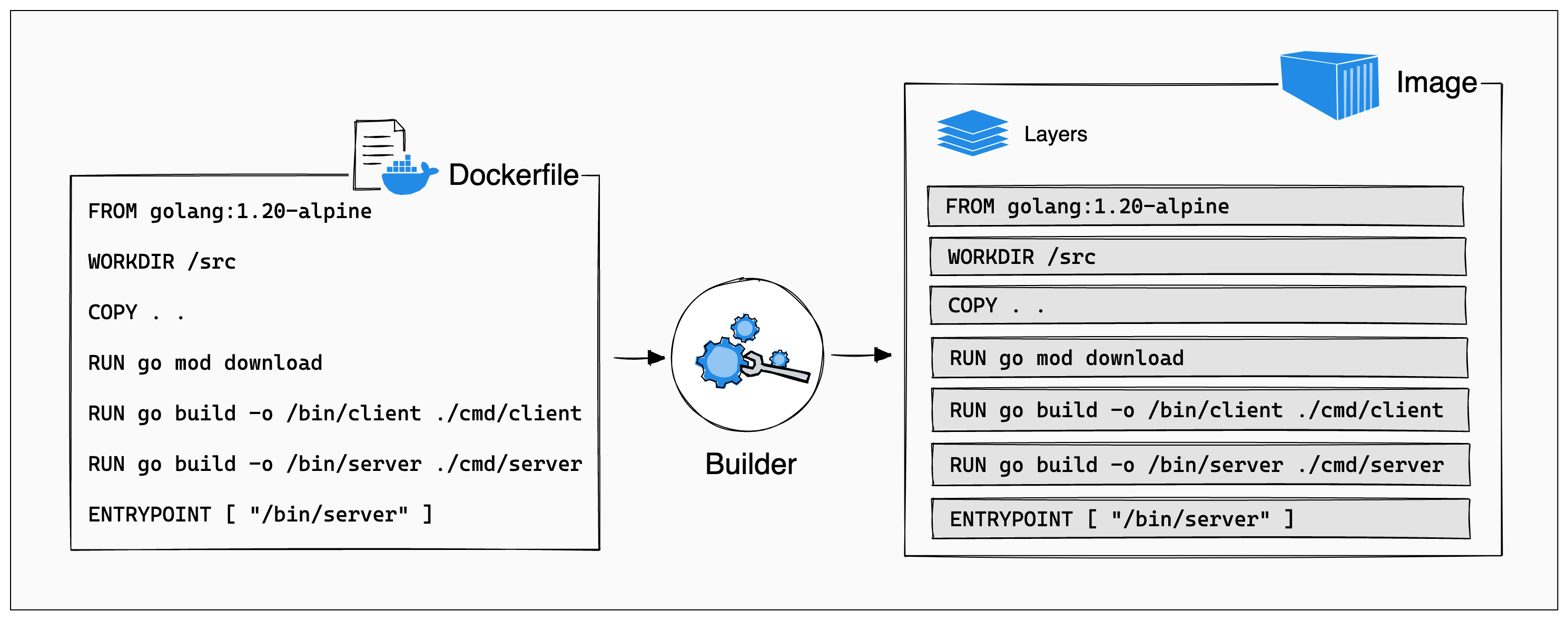
Fig. 127 Dockerfile translates into a stack of layers in a container image. Source#
Fig. 128 Busting an expensive cached layer (left). Cache optimized version (right).#
Listing the built image:
!docker image ls
REPOSITORY TAG IMAGE ID CREATED SIZE
okt/simple-fastapi latest 67acde1baf94 About an hour ago 173MB
ubuntu latest da935f064913 2 weeks ago 69.3MB
hello-world latest ee301c921b8a 7 months ago 9.14kB
Port mapping#
Note that the app runs in port 0.0.0.0:80
inside the container. We will expose this to our local machine by port mapping it to localhost:8000
. Running the image in detached mode:
!docker run -d -p 8000:80 --name fastapi okt/simple-fastapi:latest
15692290b3f217c0adee9ad011c3e268f3ab769430413e0c2bca60ad4740b883
!docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
15692290b3f2 okt/simple-fastapi:latest "uvicorn src.main:ap…" 2 seconds ago Up Less than a second 0.0.0.0:8000->80/tcp fastapi
Trying it out:
!http :8000
HTTP/1.1 200 OK
content-length: 26
content-type: application/json
date: Wed, 27 Dec 2023 23:26:06 GMT
server: uvicorn
{
"message": "Hello world!"
}
Dev environment#
This section deals with quality of life improvement for developing with Docker. For example, any changes to main.py
will not affect the running app (which is isolated). This can make development difficult with time-consuming rebuilds.
Also, if we have a large files or builds that will be used for development, then we will have to copy or rebuild this each time the image is built. Our local IDE also does not have features like autocomplete and will generally compain of missing packages not installed in the local environment.
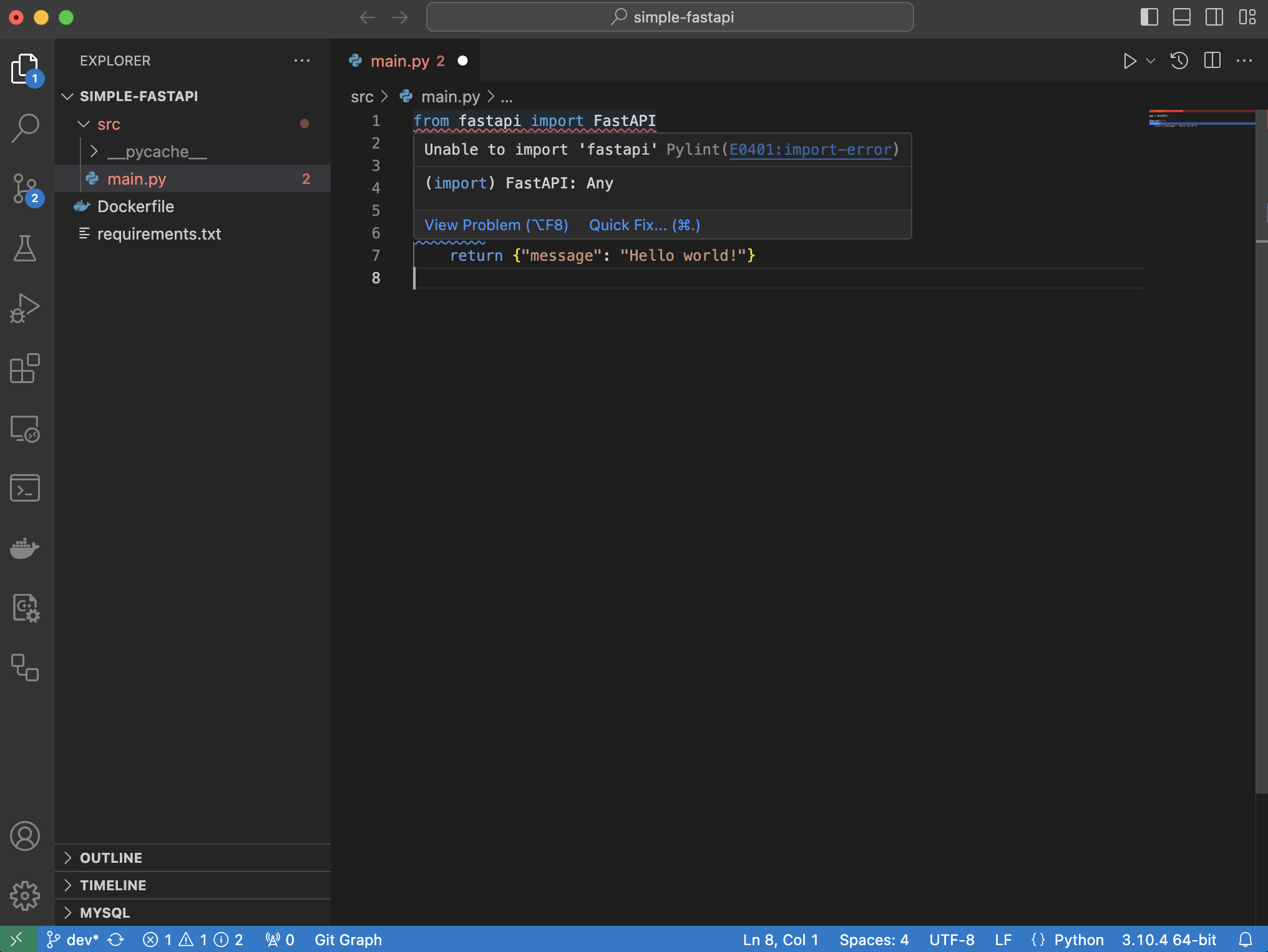
Fig. 129 Module not found. One solution is to install the requirements in a virtual env. But this is not ideal if you want straightforward reproducibility.#
Volumes#
The issue with code changes and data is fixed by using volumes. This will allow changes in the local filesystem to be reflected within the container (since files are mirrored between the two directories). In our case, the --reload
flag is essential to avoid manually restarting the uvicorn server inside the container.
!tree $(pwd)/simple-fastapi -I __pycache__
/Users/particle1331/code/ok-transformer/docs/nb/notes/containers/simple-fastapi
├── Dockerfile
├── requirements.txt
└── src
└── main.py
2 directories, 3 files
Running docker run
with -v
flag for mapping volumes and a command argument --reload
which is appended to the entrypoint. Note the --reload
flag is not in the Dockerfile
since this is not suitable for prod.
!docker rm -f fastapi >> /dev/null # delete prev container
!docker run -d -p 8000:80 -v $(pwd)/simple-fastapi:/code --name fastapi okt/simple-fastapi:latest --reload
Modifying the main file:
%%writefile ./simple-fastapi/src/main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def root():
return {"message": "Hello world + 123!"} # (!)
Overwriting ./simple-fastapi/src/main.py
Logs show that the application is reloading:
!docker logs fastapi
INFO: Will watch for changes in these directories: ['/code']
INFO: Uvicorn running on http://0.0.0.0:80 (Press CTRL+C to quit)
INFO: Started reloader process [1] using StatReload
INFO: Started server process [8]
INFO: Waiting for application startup.
INFO: Application startup complete.
The response should change without rebuild (the ff. uses httpie):
!http :8000
HTTP/1.1 200 OK
content-length: 32
content-type: application/json
date: Wed, 27 Dec 2023 23:26:16 GMT
server: uvicorn
{
"message": "Hello world + 123!"
}
Remote container IDE#
To follow this section, you have to install Docker and Dev Containers extensions in VS Code. To use an IDE with a running container, you can click on the lower left button or press CTRL+SHIFT+P and type “Dev Containers: Attach to running container”. This opens up a new window. You have to install the Python extension once to get IDE features. The experience is the same as when you SSH into a remote server.
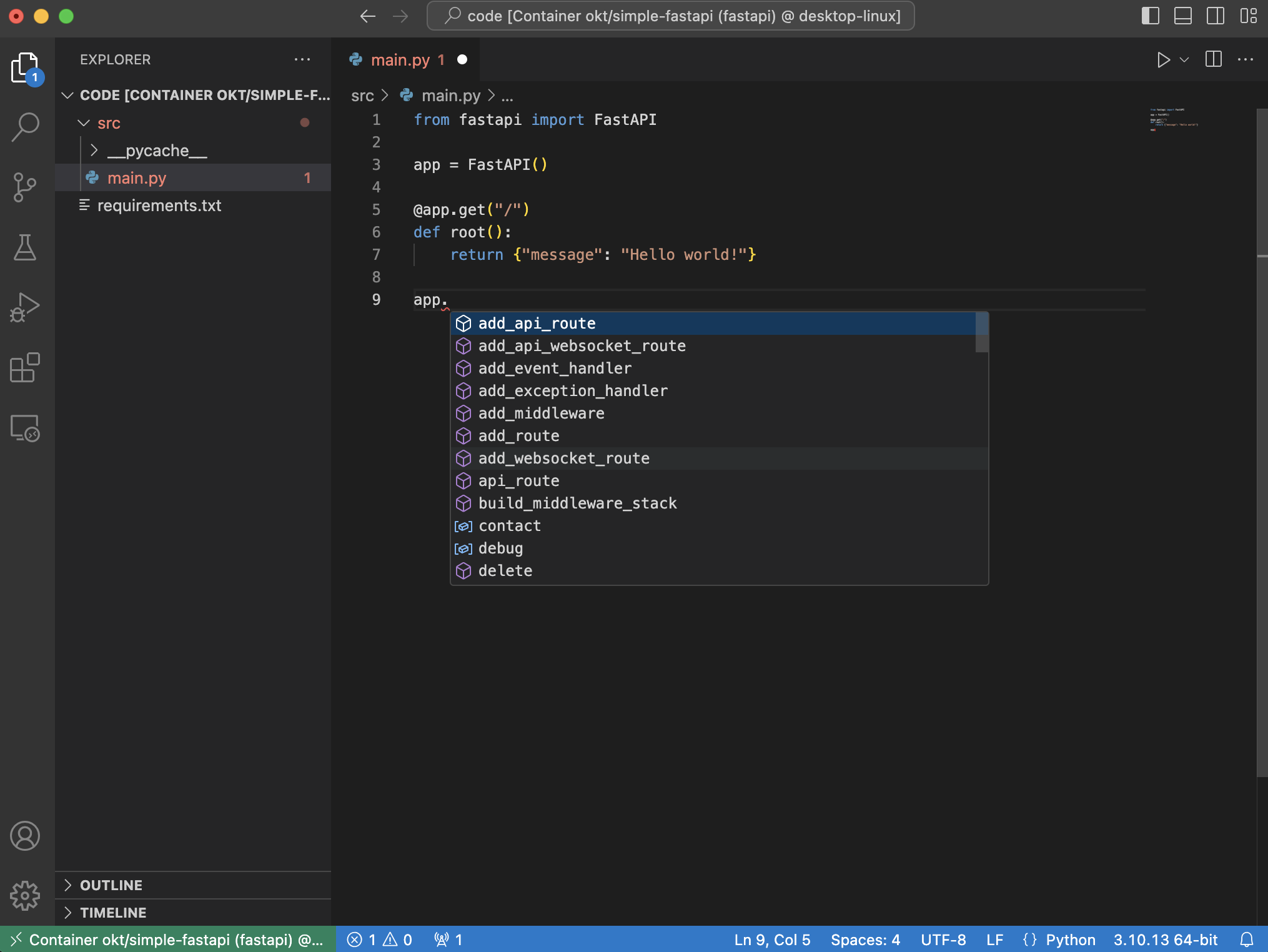
Fig. 130 IDE attached to the container with code completion and other useful features. If the container is mapped to a volume, then any change made using the IDE is mirrored in the host directory.#
Debugging#
Note: Refer to the following section on docker compose application used here.
For debugging, we use microsoft/debugpy. Run the application defined in the following compose file. This overrides the Dockerfile entrypoint and simply runs the uvicorn server with debugpy client listening on port 5678.
# containers/compose/docker-compose.debug.yml
version: "3"
services:
fastapi-server:
build: app
restart: on-failure
entrypoint: ""
command: ["sh", "-c", "pip install debugpy -t /tmp && python /tmp/debugpy --wait-for-client --listen 0.0.0.0:5678 -m uvicorn src.main:app --host 0.0.0.0 --port 8000 --reload"]
ports:
- 8080:8000
- 5678:5678
volumes:
- ./app:/code
depends_on:
- redis
redis:
image: redis:alpine
To run this:
docker compose -f docker-compose.debug.yml up
Remote attach to the FastAPI container using VS Code. In the 🐞 tab of the remote IDE, click “create a launch.json file”. Select “Remote Attach” and enter “localhost” with port “5678”. Start the debugger and add breakpoints. Then, we can make the relevant request to debug it (Fig. 131).
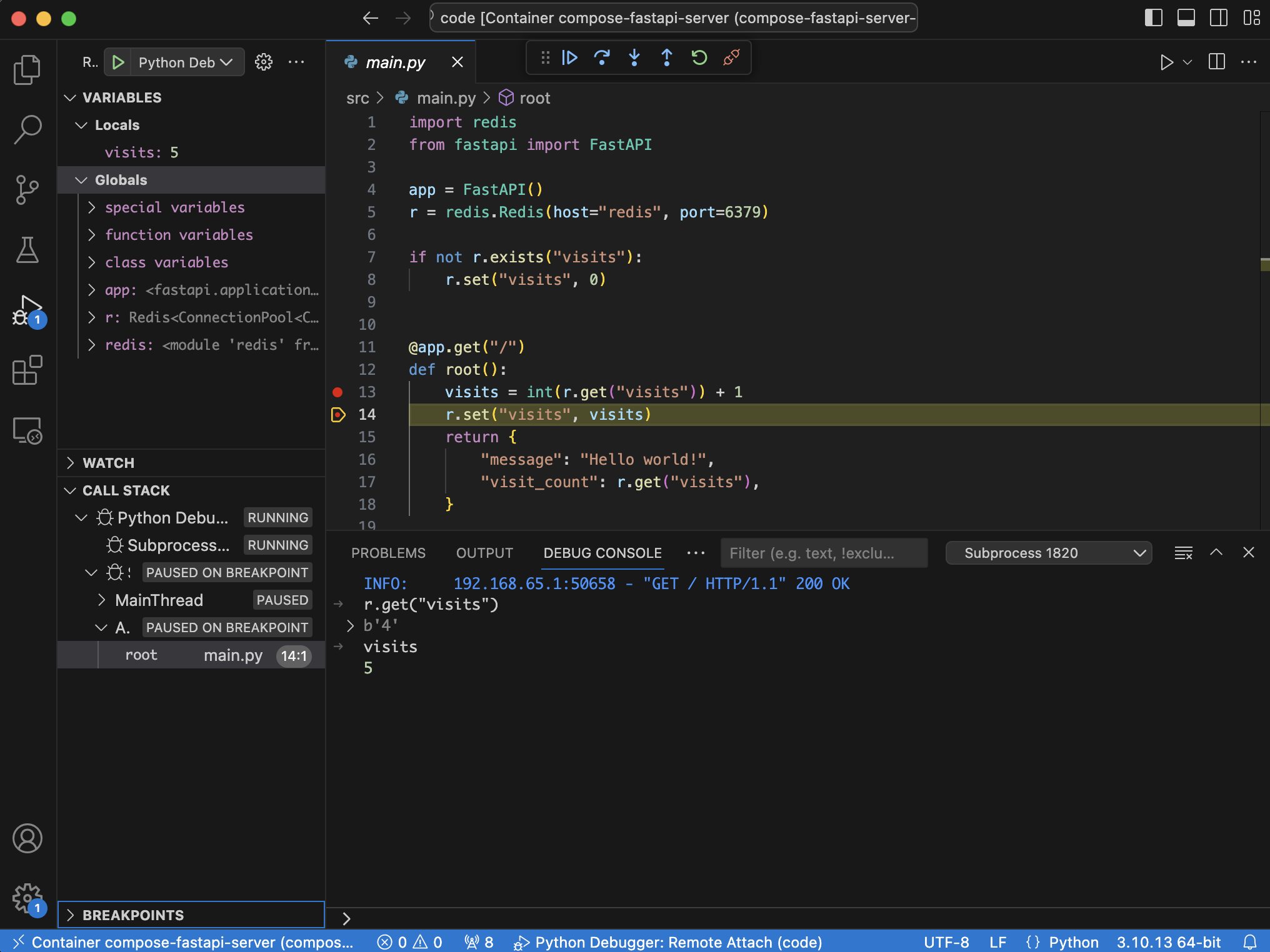
Fig. 131 Debugger running. Breakpoints are triggered after a GET request to localhost:8080
.#
Docker compose#
Multi-container applications require configuring setup and tear down of run and builds, volumes, as well as networking between multiple services. This can be tedious to do using Docker CLI commands especially during development. Docker Compose allows us to collect all configurations in a YAML file. This takes care of networking between containers as well as logging and status monitors for the whole ensemble.
Files#
The web server is in its own directory containing its corresponding Dockerfile:
!tree ./compose -I __pycache__
./compose
├── app
│ ├── Dockerfile
│ ├── requirements.txt
│ └── src
│ └── main.py
└── docker-compose.yml
3 directories, 4 files
This simply tracks the visit count along with a message. For storing the counts, we will use a redis database. Note that since compose takes care of networking, it suffices to use the container name (see compose file below) as host for the redis client:
!pygmentize ./compose/app/src/main.py
import redis
from fastapi import FastAPI
app = FastAPI()
r = redis.Redis(host="redis", port=6379)
if not r.exists("visits"):
r.set("visits", 0)
@app.get("/")
def root():
visits = int(r.get("visits")) + 1
r.set("visits", visits)
return {
"message": "Hello world!",
"visit_count": r.get("visits"),
}
Note that paths in Dockerfile and compose files are relative:
!pygmentize ./compose/app/Dockerfile
FROM python:3.10-slim
WORKDIR /code
COPY ./requirements.txt ./
RUN pip install --no-cache-dir --upgrade -r requirements.txt
COPY ./src ./src
ENTRYPOINT ["uvicorn", "src.main:app"]
The compose file simply lists the services and its run configurations. Notice that port and volume mapping are already specified here,
as well as run commands. Moreover, the dependence of the web server to the database is stated. This means the redis
service is started first.
!pygmentize ./compose/docker-compose.yml
version: "3"
services:
fastapi-server:
build: app
restart: on-failure
command: "--host 0.0.0.0 --port 80 --reload"
ports:
- 8080:80
- 5678:5678
volumes:
- ./app:/code
depends_on:
- redis
redis:
image: redis:alpine
Remark. Docker compose is typically used for development and automated testing use cases. So it’s okay to have --reload
hard coded here.
Compose up#
Starting the multi-container application. The --build
flag is optional and is used to rebuild containers from images. Again, we use -d
to run it in detached mode. Here, we also change the build context. Otherwise, we would need to add -f PATH
to point to the path of the compose file each time we use docker compose
.
os.chdir("./compose")
!docker-compose up -d --build
Show code cell output
?25l[+] Running 0/0
⠙ redis Pulling 0.1s
?25h?25l[+] Running 0/1
⠹ redis Pulling 0.2s
?25h?25l[+] Running 0/1
⠸ redis Pulling 0.3s
?25h?25l[+] Running 0/1
⠼ redis Pulling 0.4s
?25h?25l[+] Running 0/1
⠴ redis Pulling 0.5s
?25h?25l[+] Running 0/1
⠦ redis Pulling 0.6s
?25h?25l[+] Running 0/1
⠧ redis Pulling 0.7s
?25h?25l[+] Running 0/1
⠇ redis Pulling 0.8s
?25h?25l[+] Running 0/1
⠏ redis Pulling 0.9s
?25h?25l[+] Running 0/1
⠋ redis Pulling 1.0s
?25h?25l[+] Running 0/1
⠙ redis Pulling 1.1s
?25h?25l[+] Running 0/1
⠹ redis Pulling 1.2s
?25h?25l[+] Running 0/1
⠸ redis Pulling 1.3s
?25h?25l[+] Running 0/1
⠼ redis Pulling 1.4s
?25h?25l[+] Running 0/1
⠴ redis Pulling 1.5s
?25h?25l[+] Running 0/1
⠦ redis Pulling 1.6s
?25h?25l[+] Running 0/1
⠧ redis Pulling 1.7s
?25h?25l[+] Running 0/1
⠇ redis Pulling 1.8s
?25h?25l[+] Running 0/1
⠏ redis Pulling 1.9s
?25h?25l[+] Running 0/1
⠋ redis Pulling 2.0s
?25h?25l[+] Running 0/1
⠙ redis Pulling 2.1s
?25h?25l[+] Running 0/1
⠹ redis Pulling 2.2s
?25h?25l[+] Running 0/1
⠸ redis Pulling 2.3s
?25h?25l[+] Running 0/1
⠼ redis Pulling 2.4s
?25h?25l[+] Running 0/1
⠴ redis Pulling 2.5s
?25h?25l[+] Running 0/1
⠦ redis Pulling 2.6s
?25h?25l[+] Running 0/1
⠧ redis Pulling 2.7s
?25h?25l[+] Running 0/1
⠇ redis Pulling 2.8s
?25h?25l[+] Running 0/1
⠏ redis Pulling 2.9s
?25h?25l[+] Running 0/1
⠋ redis Pulling 3.0s
?25h?25l[+] Running 0/1
⠙ redis Pulling 3.1s
?25h?25l[+] Running 0/1
⠹ redis Pulling 3.2s
?25h?25l[+] Running 0/1
⠸ redis Pulling 3.3s
?25h?25l[+] Running 0/1
⠼ redis Pulling 3.4s
?25h?25l[+] Running 0/1
⠴ redis Pulling 3.5s
?25h?25l[+] Running 0/1
⠦ redis Pulling 3.6s
?25h?25l[+] Running 0/1
⠧ redis Pulling 3.7s
?25h?25l[+] Running 0/1
⠇ redis Pulling 3.8s
?25h?25l[+] Running 0/1
⠏ redis Pulling 3.9s
?25h?25l[+] Running 0/1
⠋ redis Pulling 4.0s
?25h?25l[+] Running 0/1
⠙ redis Pulling 4.1s
?25h?25l[+] Running 0/1
⠹ redis Pulling 4.2s
?25h?25l[+] Running 0/1
⠸ redis Pulling 4.3s
?25h?25l[+] Running 0/1
⠼ redis Pulling 4.4s
?25h?25l[+] Running 0/1
⠴ redis Pulling 4.5s
?25h?25l[+] Running 0/1
⠦ redis Pulling 4.6s
?25h?25l[+] Running 0/1
⠧ redis Pulling 4.7s
?25h?25l[+] Running 0/1
⠇ redis Pulling 4.8s
?25h?25l[+] Running 0/1
⠏ redis Pulling 4.9s
?25h?25l[+] Running 0/1
⠋ redis Pulling 5.0s
?25h?25l[+] Running 0/1
⠙ redis Pulling 5.1s
?25h?25l[+] Running 0/1
⠹ redis Pulling 5.2s
?25h?25l[+] Running 0/1
⠸ redis Pulling 5.3s
?25h?25l[+] Running 0/1
⠼ redis Pulling 5.4s
?25h?25l[+] Running 0/1
⠴ redis Pulling 5.5s
⠋ c30352492317 Pulling fs layer 0.1s
⠋ 2e707cb76875 Pulling fs layer 0.1s
⠋ 41a20ff430bc Pulling fs layer 0.1s
⠋ d326b14c5477 Waiting 0.1s
⠋ 00477ff4bbe5 Waiting 0.1s
⠋ 970a5a4847d9 Pulling fs layer 0.1s
⠋ 4f4fb700ef54 Waiting 0.1s
⠋ f1bbb1bc1e67 Waiting 0.1s
?25h?25l[+] Running 0/9
⠦ redis Pulling 5.6s
⠙ c30352492317 Pulling fs layer 0.2s
⠙ 2e707cb76875 Pulling fs layer 0.2s
⠙ 41a20ff430bc Pulling fs layer 0.2s
⠙ d326b14c5477 Waiting 0.2s
⠙ 00477ff4bbe5 Waiting 0.2s
⠙ 970a5a4847d9 Pulling fs layer 0.2s
⠙ 4f4fb700ef54 Waiting 0.2s
⠙ f1bbb1bc1e67 Waiting 0.2s
?25h?25l[+] Running 0/9
⠧ redis Pulling 5.7s
⠹ c30352492317 Pulling fs layer 0.3s
⠹ 2e707cb76875 Pulling fs layer 0.3s
⠹ 41a20ff430bc Pulling fs layer 0.3s
⠹ d326b14c5477 Waiting 0.3s
⠹ 00477ff4bbe5 Waiting 0.3s
⠹ 970a5a4847d9 Pulling fs layer 0.3s
⠹ 4f4fb700ef54 Waiting 0.3s
⠹ f1bbb1bc1e67 Waiting 0.3s
?25h?25l[+] Running 0/9
⠇ redis Pulling 5.8s
⠸ c30352492317 Pulling fs layer 0.4s
⠸ 2e707cb76875 Pulling fs layer 0.4s
⠸ 41a20ff430bc Pulling fs layer 0.4s
⠸ d326b14c5477 Waiting 0.4s
⠸ 00477ff4bbe5 Waiting 0.4s
⠸ 970a5a4847d9 Pulling fs layer 0.4s
⠸ 4f4fb700ef54 Waiting 0.4s
⠸ f1bbb1bc1e67 Waiting 0.4s
?25h?25l[+] Running 0/9
⠏ redis Pulling 5.9s
⠼ c30352492317 Pulling fs layer 0.5s
⠼ 2e707cb76875 Pulling fs layer 0.5s
⠼ 41a20ff430bc Pulling fs layer 0.5s
⠼ d326b14c5477 Waiting 0.5s
⠼ 00477ff4bbe5 Waiting 0.5s
⠼ 970a5a4847d9 Pulling fs layer 0.5s
⠼ 4f4fb700ef54 Waiting 0.5s
⠼ f1bbb1bc1e67 Waiting 0.5s
?25h?25l[+] Running 0/9
⠋ redis Pulling 6.0s
⠴ c30352492317 Pulling fs layer 0.6s
⠴ 2e707cb76875 Pulling fs layer 0.6s
⠴ 41a20ff430bc Pulling fs layer 0.6s
⠴ d326b14c5477 Waiting 0.6s
⠴ 00477ff4bbe5 Waiting 0.6s
⠴ 970a5a4847d9 Pulling fs layer 0.6s
⠴ 4f4fb700ef54 Waiting 0.6s
⠴ f1bbb1bc1e67 Waiting 0.6s
?25h?25l[+] Running 0/9
⠙ redis Pulling 6.1s
⠦ c30352492317 Pulling fs layer 0.7s
⠦ 2e707cb76875 Pulling fs layer 0.7s
⠦ 41a20ff430bc Pulling fs layer 0.7s
⠦ d326b14c5477 Waiting 0.7s
⠦ 00477ff4bbe5 Waiting 0.7s
⠦ 970a5a4847d9 Pulling fs layer 0.7s
⠦ 4f4fb700ef54 Waiting 0.7s
⠦ f1bbb1bc1e67 Waiting 0.7s
?25h?25l[+] Running 0/9
⠹ redis Pulling 6.2s
⠧ c30352492317 Pulling fs layer 0.8s
⠧ 2e707cb76875 Pulling fs layer 0.8s
⠧ 41a20ff430bc Pulling fs layer 0.8s
⠧ d326b14c5477 Waiting 0.8s
⠧ 00477ff4bbe5 Waiting 0.8s
⠧ 970a5a4847d9 Pulling fs layer 0.8s
⠧ 4f4fb700ef54 Waiting 0.8s
⠧ f1bbb1bc1e67 Waiting 0.8s
?25h?25l[+] Running 0/9
⠸ redis Pulling 6.3s
⠇ c30352492317 Pulling fs layer 0.9s
⠇ 2e707cb76875 Pulling fs layer 0.9s
⠇ 41a20ff430bc Pulling fs layer 0.9s
⠇ d326b14c5477 Waiting 0.9s
⠇ 00477ff4bbe5 Waiting 0.9s
⠇ 970a5a4847d9 Pulling fs layer 0.9s
⠇ 4f4fb700ef54 Waiting 0.9s
⠇ f1bbb1bc1e67 Waiting 0.9s
?25h?25l[+] Running 0/9
⠼ redis Pulling 6.4s
⠏ c30352492317 Pulling fs layer 1.0s
⠏ 2e707cb76875 Pulling fs layer 1.0s
⠏ 41a20ff430bc Pulling fs layer 1.0s
⠏ d326b14c5477 Waiting 1.0s
⠏ 00477ff4bbe5 Waiting 1.0s
⠏ 970a5a4847d9 Pulling fs layer 1.0s
⠏ 4f4fb700ef54 Waiting 1.0s
⠏ f1bbb1bc1e67 Waiting 1.0s
?25h?25l[+] Running 0/9
⠴ redis Pulling 6.5s
⠋ c30352492317 Pulling fs layer 1.1s
⠋ 2e707cb76875 Pulling fs layer 1.1s
⠋ 41a20ff430bc Pulling fs layer 1.1s
⠋ d326b14c5477 Waiting 1.1s
⠋ 00477ff4bbe5 Waiting 1.1s
⠋ 970a5a4847d9 Pulling fs layer 1.1s
⠋ 4f4fb700ef54 Waiting 1.1s
⠋ f1bbb1bc1e67 Waiting 1.1s
?25h?25l[+] Running 0/9
⠦ redis Pulling 6.6s
⠙ c30352492317 Pulling fs layer 1.2s
⠙ 2e707cb76875 Pulling fs layer 1.2s
⠙ 41a20ff430bc Pulling fs layer 1.2s
⠙ d326b14c5477 Waiting 1.2s
⠙ 00477ff4bbe5 Waiting 1.2s
⠙ 970a5a4847d9 Pulling fs layer 1.2s
⠙ 4f4fb700ef54 Waiting 1.2s
⠙ f1bbb1bc1e67 Waiting 1.2s
?25h?25l[+] Running 0/9
⠧ redis Pulling 6.7s
⠹ c30352492317 Pulling fs layer 1.3s
⠹ 2e707cb76875 Pulling fs layer 1.3s
⠹ 41a20ff430bc Pulling fs layer 1.3s
⠹ d326b14c5477 Waiting 1.3s
⠹ 00477ff4bbe5 Waiting 1.3s
⠹ 970a5a4847d9 Pulling fs layer 1.3s
⠹ 4f4fb700ef54 Waiting 1.3s
⠹ f1bbb1bc1e67 Waiting 1.3s
?25h?25l[+] Running 0/9
⠇ redis Pulling 6.8s
⠸ c30352492317 Pulling fs layer 1.4s
⠸ 2e707cb76875 Pulling fs layer 1.4s
⠸ 41a20ff430bc Pulling fs layer 1.4s
⠸ d326b14c5477 Waiting 1.4s
⠸ 00477ff4bbe5 Waiting 1.4s
⠸ 970a5a4847d9 Pulling fs layer 1.4s
⠸ 4f4fb700ef54 Waiting 1.4s
⠸ f1bbb1bc1e67 Waiting 1.4s
?25h?25l[+] Running 0/9
⠏ redis Pulling 6.9s
⠼ c30352492317 Pulling fs layer 1.5s
⠼ 2e707cb76875 Pulling fs layer 1.5s
⠼ 41a20ff430bc Pulling fs layer 1.5s
⠼ d326b14c5477 Waiting 1.5s
⠼ 00477ff4bbe5 Waiting 1.5s
⠼ 970a5a4847d9 Pulling fs layer 1.5s
⠼ 4f4fb700ef54 Waiting 1.5s
⠼ f1bbb1bc1e67 Waiting 1.5s
?25h?25l[+] Running 0/9
⠋ redis Pulling 7.0s
⠴ c30352492317 Pulling fs layer 1.6s
⠴ 2e707cb76875 Pulling fs layer 1.6s
⠴ 41a20ff430bc Pulling fs layer 1.6s
⠴ d326b14c5477 Waiting 1.6s
⠴ 00477ff4bbe5 Waiting 1.6s
⠴ 970a5a4847d9 Pulling fs layer 1.6s
⠴ 4f4fb700ef54 Waiting 1.6s
⠴ f1bbb1bc1e67 Waiting 1.6s
?25h?25l[+] Running 0/9
⠙ redis Pulling 7.1s
⠦ c30352492317 Pulling fs layer 1.7s
⠦ 2e707cb76875 Pulling fs layer 1.7s
⠦ 41a20ff430bc Pulling fs layer 1.7s
⠦ d326b14c5477 Waiting 1.7s
⠦ 00477ff4bbe5 Waiting 1.7s
⠦ 970a5a4847d9 Pulling fs layer 1.7s
⠦ 4f4fb700ef54 Waiting 1.7s
⠦ f1bbb1bc1e67 Waiting 1.7s
?25h?25l[+] Running 0/9
⠹ redis Pulling 7.2s
⠧ c30352492317 Pulling fs layer 1.8s
⠧ 2e707cb76875 Pulling fs layer 1.8s
⠧ 41a20ff430bc Pulling fs layer 1.8s
⠧ d326b14c5477 Waiting 1.8s
⠧ 00477ff4bbe5 Waiting 1.8s
⠧ 970a5a4847d9 Pulling fs layer 1.8s
⠧ 4f4fb700ef54 Waiting 1.8s
⠧ f1bbb1bc1e67 Waiting 1.8s
?25h?25l[+] Running 0/9
⠸ redis Pulling 7.3s
⠇ c30352492317 Downloading 34.14kB/3.348MB 1.9s
⠇ 2e707cb76875 Download complete 1.9s
⠇ 41a20ff430bc Downloading 4.107kB/345kB 1.9s
⠇ d326b14c5477 Waiting 1.9s
⠇ 00477ff4bbe5 Waiting 1.9s
⠇ 970a5a4847d9 Pulling fs layer 1.9s
⠇ 4f4fb700ef54 Waiting 1.9s
⠇ f1bbb1bc1e67 Waiting 1.9s
?25h?25l[+] Running 0/9
⠼ redis Pulling 7.4s
⠏ c30352492317 Downloading 143.8kB/3.348MB 2.0s
⠏ 2e707cb76875 Download complete 2.0s
⠏ 41a20ff430bc Downloading 4.107kB/345kB 2.0s
⠏ d326b14c5477 Waiting 2.0s
⠏ 00477ff4bbe5 Waiting 2.0s
⠏ 970a5a4847d9 Pulling fs layer 2.0s
⠏ 4f4fb700ef54 Waiting 2.0s
⠏ f1bbb1bc1e67 Waiting 2.0s
?25h?25l[+] Running 0/9
⠴ redis Pulling 7.5s
⠋ c30352492317 Downloading 143.8kB/3.348MB 2.1s
⠋ 2e707cb76875 Download complete 2.1s
⠋ 41a20ff430bc Downloading 135.3kB/345kB 2.1s
⠋ d326b14c5477 Waiting 2.1s
⠋ 00477ff4bbe5 Waiting 2.1s
⠋ 970a5a4847d9 Pulling fs layer 2.1s
⠋ 4f4fb700ef54 Waiting 2.1s
⠋ f1bbb1bc1e67 Waiting 2.1s
?25h?25l[+] Running 0/9
⠦ redis Pulling 7.6s
⠙ c30352492317 Downloading 262.1kB/3.348MB 2.2s
⠙ 2e707cb76875 Download complete 2.2s
⠙ 41a20ff430bc Downloading 135.3kB/345kB 2.2s
⠙ d326b14c5477 Waiting 2.2s
⠙ 00477ff4bbe5 Waiting 2.2s
⠙ 970a5a4847d9 Pulling fs layer 2.2s
⠙ 4f4fb700ef54 Waiting 2.2s
⠙ f1bbb1bc1e67 Waiting 2.2s
?25h?25l[+] Running 0/9
⠧ redis Pulling 7.7s
⠹ c30352492317 Downloading 262.1kB/3.348MB 2.3s
⠹ 2e707cb76875 Download complete 2.3s
⠹ 41a20ff430bc Downloading 135.3kB/345kB 2.3s
⠹ d326b14c5477 Waiting 2.3s
⠹ 00477ff4bbe5 Waiting 2.3s
⠹ 970a5a4847d9 Pulling fs layer 2.3s
⠹ 4f4fb700ef54 Waiting 2.3s
⠹ f1bbb1bc1e67 Waiting 2.3s
?25h?25l[+] Running 0/9
⠇ redis Pulling 7.8s
⠸ c30352492317 Downloading 606.2kB/3.348MB 2.4s
⠸ 2e707cb76875 Download complete 2.4s
⠸ 41a20ff430bc Download complete 2.4s
⠸ d326b14c5477 Waiting 2.4s
⠸ 00477ff4bbe5 Waiting 2.4s
⠸ 970a5a4847d9 Pulling fs layer 2.4s
⠸ 4f4fb700ef54 Waiting 2.4s
⠸ f1bbb1bc1e67 Waiting 2.4s
?25h?25l[+] Running 0/9
⠏ redis Pulling 7.9s
⠼ c30352492317 Downloading 852kB/3.348MB 2.5s
⠼ 2e707cb76875 Download complete 2.5s
⠼ 41a20ff430bc Download complete 2.5s
⠼ d326b14c5477 Waiting 2.5s
⠼ 00477ff4bbe5 Waiting 2.5s
⠼ 970a5a4847d9 Pulling fs layer 2.5s
⠼ 4f4fb700ef54 Waiting 2.5s
⠼ f1bbb1bc1e67 Waiting 2.5s
?25h?25l[+] Running 0/9
⠋ redis Pulling 8.0s
⠴ c30352492317 Downloading 852kB/3.348MB 2.6s
⠴ 2e707cb76875 Download complete 2.6s
⠴ 41a20ff430bc Download complete 2.6s
⠴ d326b14c5477 Waiting 2.6s
⠴ 00477ff4bbe5 Waiting 2.6s
⠴ 970a5a4847d9 Pulling fs layer 2.6s
⠴ 4f4fb700ef54 Waiting 2.6s
⠴ f1bbb1bc1e67 Waiting 2.6s
?25h?25l[+] Running 0/9
⠙ redis Pulling 8.1s
⠦ c30352492317 Downloading 901.1kB/3.348MB 2.7s
⠦ 2e707cb76875 Download complete 2.7s
⠦ 41a20ff430bc Download complete 2.7s
⠦ d326b14c5477 Waiting 2.7s
⠦ 00477ff4bbe5 Waiting 2.7s
⠦ 970a5a4847d9 Pulling fs layer 2.7s
⠦ 4f4fb700ef54 Waiting 2.7s
⠦ f1bbb1bc1e67 Waiting 2.7s
?25h?25l[+] Running 0/9
⠹ redis Pulling 8.2s
⠧ c30352492317 Downloading 1.436MB/3.348MB 2.8s
⠧ 2e707cb76875 Download complete 2.8s
⠧ 41a20ff430bc Download complete 2.8s
⠧ d326b14c5477 Waiting 2.8s
⠧ 00477ff4bbe5 Waiting 2.8s
⠧ 970a5a4847d9 Pulling fs layer 2.8s
⠧ 4f4fb700ef54 Waiting 2.8s
⠧ f1bbb1bc1e67 Waiting 2.8s
?25h?25l[+] Running 0/9
⠸ redis Pulling 8.3s
⠇ c30352492317 Downloading 1.436MB/3.348MB 2.9s
⠇ 2e707cb76875 Download complete 2.9s
⠇ 41a20ff430bc Download complete 2.9s
⠇ d326b14c5477 Waiting 2.9s
⠇ 00477ff4bbe5 Waiting 2.9s
⠇ 970a5a4847d9 Pulling fs layer 2.9s
⠇ 4f4fb700ef54 Waiting 2.9s
⠇ f1bbb1bc1e67 Waiting 2.9s
?25h?25l[+] Running 0/9
⠼ redis Pulling 8.4s
⠏ c30352492317 Downloading 1.731MB/3.348MB 3.0s
⠏ 2e707cb76875 Download complete 3.0s
⠏ 41a20ff430bc Download complete 3.0s
⠏ d326b14c5477 Waiting 3.0s
⠏ 00477ff4bbe5 Waiting 3.0s
⠏ 970a5a4847d9 Pulling fs layer 3.0s
⠏ 4f4fb700ef54 Waiting 3.0s
⠏ f1bbb1bc1e67 Waiting 3.0s
?25h?25l[+] Running 0/9
⠴ redis Pulling 8.5s
⠋ c30352492317 Downloading 2.259MB/3.348MB 3.1s
⠋ 2e707cb76875 Download complete 3.1s
⠋ 41a20ff430bc Download complete 3.1s
⠋ d326b14c5477 Waiting 3.1s
⠋ 00477ff4bbe5 Waiting 3.1s
⠋ 970a5a4847d9 Pulling fs layer 3.1s
⠋ 4f4fb700ef54 Waiting 3.1s
⠋ f1bbb1bc1e67 Waiting 3.1s
?25h?25l[+] Running 0/9
⠦ redis Pulling 8.6s
⠙ c30352492317 Downloading 2.702MB/3.348MB 3.2s
⠙ 2e707cb76875 Download complete 3.2s
⠙ 41a20ff430bc Download complete 3.2s
⠙ d326b14c5477 Waiting 3.2s
⠙ 00477ff4bbe5 Waiting 3.2s
⠙ 970a5a4847d9 Pulling fs layer 3.2s
⠙ 4f4fb700ef54 Waiting 3.2s
⠙ f1bbb1bc1e67 Waiting 3.2s
?25h?25l[+] Running 0/9
⠧ redis Pulling 8.7s
⠹ c30352492317 Downloading 3.046MB/3.348MB 3.3s
⠹ 2e707cb76875 Download complete 3.3s
⠹ 41a20ff430bc Download complete 3.3s
⠹ d326b14c5477 Waiting 3.3s
⠹ 00477ff4bbe5 Waiting 3.3s
⠹ 970a5a4847d9 Pulling fs layer 3.3s
⠹ 4f4fb700ef54 Waiting 3.3s
⠹ f1bbb1bc1e67 Waiting 3.3s
?25h?25l[+] Running 0/9
⠇ redis Pulling 8.8s
⠸ c30352492317 Downloading 3.144MB/3.348MB 3.4s
⠸ 2e707cb76875 Download complete 3.4s
⠸ 41a20ff430bc Download complete 3.4s
⠸ d326b14c5477 Waiting 3.4s
⠸ 00477ff4bbe5 Waiting 3.4s
⠸ 970a5a4847d9 Pulling fs layer 3.4s
⠸ 4f4fb700ef54 Waiting 3.4s
⠸ f1bbb1bc1e67 Waiting 3.4s
?25h?25l[+] Running 0/9
⠏ redis Pulling 8.9s
⠼ c30352492317 Extracting 65.54kB/3.348MB 3.5s
⠼ 2e707cb76875 Download complete 3.5s
⠼ 41a20ff430bc Download complete 3.5s
⠼ d326b14c5477 Waiting 3.5s
⠼ 00477ff4bbe5 Waiting 3.5s
⠼ 970a5a4847d9 Pulling fs layer 3.5s
⠼ 4f4fb700ef54 Waiting 3.5s
⠼ f1bbb1bc1e67 Waiting 3.5s
?25h?25l[+] Running 0/9
⠋ redis Pulling 9.0s
⠴ c30352492317 Extracting 327.7kB/3.348MB 3.6s
⠴ 2e707cb76875 Download complete 3.6s
⠴ 41a20ff430bc Download complete 3.6s
⠴ d326b14c5477 Waiting 3.6s
⠴ 00477ff4bbe5 Waiting 3.6s
⠴ 970a5a4847d9 Pulling fs layer 3.6s
⠴ 4f4fb700ef54 Waiting 3.6s
⠴ f1bbb1bc1e67 Waiting 3.6s
?25h?25l[+] Running 0/9
⠙ redis Pulling 9.1s
⠦ c30352492317 Extracting 3.348MB/3.348MB 3.7s
⠦ 2e707cb76875 Download complete 3.7s
⠦ 41a20ff430bc Download complete 3.7s
⠦ d326b14c5477 Waiting 3.7s
⠦ 00477ff4bbe5 Waiting 3.7s
⠦ 970a5a4847d9 Pulling fs layer 3.7s
⠦ 4f4fb700ef54 Waiting 3.7s
⠦ f1bbb1bc1e67 Waiting 3.7s
?25h?25l[+] Running 2/9
⠹ redis Pulling 9.2s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠧ 41a20ff430bc Extracting 32.77kB/345kB 3.8s
⠧ d326b14c5477 Waiting 3.8s
⠧ 00477ff4bbe5 Waiting 3.8s
⠧ 970a5a4847d9 Pulling fs layer 3.8s
⠧ 4f4fb700ef54 Waiting 3.8s
⠧ f1bbb1bc1e67 Waiting 3.8s
?25h?25l[+] Running 2/9
⠸ redis Pulling 9.3s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠇ 41a20ff430bc Extracting 32.77kB/345kB 3.9s
⠇ d326b14c5477 Downloading 10.3kB/944.6kB 3.9s
⠇ 00477ff4bbe5 Waiting 3.9s
⠇ 970a5a4847d9 Pulling fs layer 3.9s
⠇ 4f4fb700ef54 Waiting 3.9s
⠇ f1bbb1bc1e67 Waiting 3.9s
?25h?25l[+] Running 2/9
⠼ redis Pulling 9.4s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠏ 41a20ff430bc Extracting 345kB/345kB 4.0s
⠏ d326b14c5477 Downloading 130.3kB/944.6kB 4.0s
⠏ 00477ff4bbe5 Waiting 4.0s
⠏ 970a5a4847d9 Pulling fs layer 4.0s
⠏ 4f4fb700ef54 Waiting 4.0s
⠏ f1bbb1bc1e67 Waiting 4.0s
?25h?25l[+] Running 3/9
⠴ redis Pulling 9.5s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠋ d326b14c5477 Downloading 130.3kB/944.6kB 4.1s
⠋ 00477ff4bbe5 Waiting 4.1s
⠋ 970a5a4847d9 Pulling fs layer 4.1s
⠋ 4f4fb700ef54 Waiting 4.1s
⠋ f1bbb1bc1e67 Waiting 4.1s
?25h?25l[+] Running 3/9
⠦ redis Pulling 9.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠙ d326b14c5477 Downloading 261.4kB/944.6kB 4.2s
⠙ 00477ff4bbe5 Waiting 4.2s
⠙ 970a5a4847d9 Pulling fs layer 4.2s
⠙ 4f4fb700ef54 Waiting 4.2s
⠙ f1bbb1bc1e67 Waiting 4.2s
?25h?25l[+] Running 3/9
⠧ redis Pulling 9.7s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠹ d326b14c5477 Downloading 572.7kB/944.6kB 4.3s
⠹ 00477ff4bbe5 Waiting 4.3s
⠹ 970a5a4847d9 Pulling fs layer 4.3s
⠹ 4f4fb700ef54 Waiting 4.3s
⠹ f1bbb1bc1e67 Waiting 4.3s
?25h?25l[+] Running 3/9
⠇ redis Pulling 9.8s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠸ d326b14c5477 Extracting 32.77kB/944.6kB 4.4s
⠸ 00477ff4bbe5 Waiting 4.4s
⠸ 970a5a4847d9 Pulling fs layer 4.4s
⠸ 4f4fb700ef54 Waiting 4.4s
⠸ f1bbb1bc1e67 Waiting 4.4s
?25h?25l[+] Running 4/9
⠏ redis Pulling 9.9s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠼ 00477ff4bbe5 Waiting 4.5s
⠼ 970a5a4847d9 Pulling fs layer 4.5s
⠼ 4f4fb700ef54 Waiting 4.5s
⠼ f1bbb1bc1e67 Waiting 4.5s
?25h?25l[+] Running 4/9
⠋ redis Pulling 10.0s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠴ 00477ff4bbe5 Downloading 123.7kB/12.15MB 4.6s
⠴ 970a5a4847d9 Pulling fs layer 4.6s
⠴ 4f4fb700ef54 Waiting 4.6s
⠴ f1bbb1bc1e67 Waiting 4.6s
?25h?25l[+] Running 4/9
⠙ redis Pulling 10.1s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠦ 00477ff4bbe5 Downloading 123.7kB/12.15MB 4.7s
⠦ 970a5a4847d9 Pulling fs layer 4.7s
⠦ 4f4fb700ef54 Waiting 4.7s
⠦ f1bbb1bc1e67 Waiting 4.7s
?25h?25l[+] Running 4/9
⠹ redis Pulling 10.2s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠧ 00477ff4bbe5 Downloading 250.5kB/12.15MB 4.8s
⠧ 970a5a4847d9 Pulling fs layer 4.8s
⠧ 4f4fb700ef54 Waiting 4.8s
⠧ f1bbb1bc1e67 Waiting 4.8s
?25h?25l[+] Running 4/9
⠸ redis Pulling 10.3s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠇ 00477ff4bbe5 Downloading 376.8kB/12.15MB 4.9s
⠇ 970a5a4847d9 Pulling fs layer 4.9s
⠇ 4f4fb700ef54 Waiting 4.9s
⠇ f1bbb1bc1e67 Waiting 4.9s
?25h?25l[+] Running 4/9
⠼ redis Pulling 10.4s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠏ 00477ff4bbe5 Downloading 639kB/12.15MB 5.0s
⠏ 970a5a4847d9 Verifying Checksum 5.0s
⠏ 4f4fb700ef54 Waiting 5.0s
⠏ f1bbb1bc1e67 Waiting 5.0s
?25h?25l[+] Running 4/9
⠴ redis Pulling 10.5s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠋ 00477ff4bbe5 Downloading 639kB/12.15MB 5.1s
⠋ 970a5a4847d9 Verifying Checksum 5.1s
⠋ 4f4fb700ef54 Waiting 5.1s
⠋ f1bbb1bc1e67 Waiting 5.1s
?25h?25l[+] Running 4/9
⠦ redis Pulling 10.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠙ 00477ff4bbe5 Downloading 1.032MB/12.15MB 5.2s
⠙ 970a5a4847d9 Verifying Checksum 5.2s
⠙ 4f4fb700ef54 Waiting 5.2s
⠙ f1bbb1bc1e67 Waiting 5.2s
?25h?25l[+] Running 4/9
⠧ redis Pulling 10.7s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠹ 00477ff4bbe5 Downloading 1.42MB/12.15MB 5.3s
⠹ 970a5a4847d9 Verifying Checksum 5.3s
⠹ 4f4fb700ef54 Waiting 5.3s
⠹ f1bbb1bc1e67 Waiting 5.3s
?25h?25l[+] Running 4/9
⠇ redis Pulling 10.8s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠸ 00477ff4bbe5 Downloading 1.682MB/12.15MB 5.4s
⠸ 970a5a4847d9 Verifying Checksum 5.4s
⠸ 4f4fb700ef54 Waiting 5.4s
⠸ f1bbb1bc1e67 Waiting 5.4s
?25h?25l[+] Running 4/9
⠏ redis Pulling 10.9s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠼ 00477ff4bbe5 Downloading 2.075MB/12.15MB 5.5s
⠼ 970a5a4847d9 Verifying Checksum 5.5s
⠼ 4f4fb700ef54 Waiting 5.5s
⠼ f1bbb1bc1e67 Waiting 5.5s
?25h?25l[+] Running 4/9
⠋ redis Pulling 11.0s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠴ 00477ff4bbe5 Downloading 2.468MB/12.15MB 5.6s
⠴ 970a5a4847d9 Verifying Checksum 5.6s
⠴ 4f4fb700ef54 Waiting 5.6s
⠴ f1bbb1bc1e67 Waiting 5.6s
?25h?25l[+] Running 4/9
⠙ redis Pulling 11.1s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠦ 00477ff4bbe5 Downloading 2.468MB/12.15MB 5.7s
⠦ 970a5a4847d9 Verifying Checksum 5.7s
⠦ 4f4fb700ef54 Waiting 5.7s
⠦ f1bbb1bc1e67 Waiting 5.7s
?25h?25l[+] Running 4/9
⠹ redis Pulling 11.2s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠧ 00477ff4bbe5 Downloading 2.997MB/12.15MB 5.8s
⠧ 970a5a4847d9 Verifying Checksum 5.8s
⠧ 4f4fb700ef54 Waiting 5.8s
⠧ f1bbb1bc1e67 Waiting 5.8s
?25h?25l[+] Running 4/9
⠸ redis Pulling 11.3s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠇ 00477ff4bbe5 Downloading 3.652MB/12.15MB 5.9s
⠇ 970a5a4847d9 Verifying Checksum 5.9s
⠇ 4f4fb700ef54 Waiting 5.9s
⠇ f1bbb1bc1e67 Waiting 5.9s
?25h?25l[+] Running 4/9
⠼ redis Pulling 11.4s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠏ 00477ff4bbe5 Downloading 4.18MB/12.15MB 6.0s
⠏ 970a5a4847d9 Verifying Checksum 6.0s
⠏ 4f4fb700ef54 Waiting 6.0s
⠏ f1bbb1bc1e67 Waiting 6.0s
?25h?25l[+] Running 4/9
⠴ redis Pulling 11.5s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠋ 00477ff4bbe5 Downloading 4.705MB/12.15MB 6.1s
⠋ 970a5a4847d9 Verifying Checksum 6.1s
⠋ 4f4fb700ef54 Waiting 6.1s
⠋ f1bbb1bc1e67 Waiting 6.1s
?25h?25l[+] Running 4/9
⠦ redis Pulling 11.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠙ 00477ff4bbe5 Downloading 4.967MB/12.15MB 6.2s
⠙ 970a5a4847d9 Verifying Checksum 6.2s
⠙ 4f4fb700ef54 Waiting 6.2s
⠙ f1bbb1bc1e67 Waiting 6.2s
?25h?25l[+] Running 4/9
⠧ redis Pulling 11.7s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠹ 00477ff4bbe5 Downloading 5.36MB/12.15MB 6.3s
⠹ 970a5a4847d9 Verifying Checksum 6.3s
⠹ 4f4fb700ef54 Waiting 6.3s
⠹ f1bbb1bc1e67 Waiting 6.3s
?25h?25l[+] Running 4/9
⠇ redis Pulling 11.8s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠸ 00477ff4bbe5 Downloading 5.36MB/12.15MB 6.4s
⠸ 970a5a4847d9 Verifying Checksum 6.4s
⠸ 4f4fb700ef54 Waiting 6.4s
⠸ f1bbb1bc1e67 Waiting 6.4s
?25h?25l[+] Running 4/9
⠏ redis Pulling 11.9s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠼ 00477ff4bbe5 Downloading 5.884MB/12.15MB 6.5s
⠼ 970a5a4847d9 Verifying Checksum 6.5s
⠼ 4f4fb700ef54 Waiting 6.5s
⠼ f1bbb1bc1e67 Waiting 6.5s
?25h?25l[+] Running 4/9
⠋ redis Pulling 12.0s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠴ 00477ff4bbe5 Downloading 6.138MB/12.15MB 6.6s
⠴ 970a5a4847d9 Verifying Checksum 6.6s
⠴ 4f4fb700ef54 Waiting 6.6s
⠴ f1bbb1bc1e67 Waiting 6.6s
?25h?25l[+] Running 4/9
⠙ redis Pulling 12.1s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠦ 00477ff4bbe5 Downloading 6.662MB/12.15MB 6.7s
⠦ 970a5a4847d9 Verifying Checksum 6.7s
⠦ 4f4fb700ef54 Waiting 6.7s
⠦ f1bbb1bc1e67 Waiting 6.7s
?25h?25l[+] Running 4/9
⠹ redis Pulling 12.2s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠧ 00477ff4bbe5 Downloading 7.306MB/12.15MB 6.8s
⠧ 970a5a4847d9 Verifying Checksum 6.8s
⠧ 4f4fb700ef54 Waiting 6.8s
⠧ f1bbb1bc1e67 Waiting 6.8s
?25h?25l[+] Running 4/9
⠸ redis Pulling 12.3s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠇ 00477ff4bbe5 Downloading 7.306MB/12.15MB 6.9s
⠇ 970a5a4847d9 Verifying Checksum 6.9s
⠇ 4f4fb700ef54 Waiting 6.9s
⠇ f1bbb1bc1e67 Waiting 6.9s
?25h?25l[+] Running 4/9
⠼ redis Pulling 12.4s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠏ 00477ff4bbe5 Downloading 7.306MB/12.15MB 7.0s
⠏ 970a5a4847d9 Verifying Checksum 7.0s
⠏ 4f4fb700ef54 Waiting 7.0s
⠏ f1bbb1bc1e67 Waiting 7.0s
?25h?25l[+] Running 4/9
⠴ redis Pulling 12.5s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠋ 00477ff4bbe5 Downloading 7.306MB/12.15MB 7.1s
⠋ 970a5a4847d9 Verifying Checksum 7.1s
⠋ 4f4fb700ef54 Waiting 7.1s
⠋ f1bbb1bc1e67 Waiting 7.1s
?25h?25l[+] Running 4/9
⠦ redis Pulling 12.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠙ 00477ff4bbe5 Downloading 7.306MB/12.15MB 7.2s
⠙ 970a5a4847d9 Verifying Checksum 7.2s
⠙ 4f4fb700ef54 Waiting 7.2s
⠙ f1bbb1bc1e67 Waiting 7.2s
?25h?25l[+] Running 4/9
⠧ redis Pulling 12.7s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠹ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.3s
⠹ 970a5a4847d9 Verifying Checksum 7.3s
⠹ 4f4fb700ef54 Download complete 7.3s
⠹ f1bbb1bc1e67 Waiting 7.3s
?25h?25l[+] Running 4/9
⠇ redis Pulling 12.8s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠸ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.4s
⠸ 970a5a4847d9 Verifying Checksum 7.4s
⠸ 4f4fb700ef54 Download complete 7.4s
⠸ f1bbb1bc1e67 Waiting 7.4s
?25h?25l[+] Running 4/9
⠏ redis Pulling 12.9s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠼ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.5s
⠼ 970a5a4847d9 Verifying Checksum 7.5s
⠼ 4f4fb700ef54 Download complete 7.5s
⠼ f1bbb1bc1e67 Waiting 7.5s
?25h?25l[+] Running 4/9
⠋ redis Pulling 13.0s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠴ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.6s
⠴ 970a5a4847d9 Verifying Checksum 7.6s
⠴ 4f4fb700ef54 Download complete 7.6s
⠴ f1bbb1bc1e67 Waiting 7.6s
?25h?25l[+] Running 4/9
⠙ redis Pulling 13.1s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠦ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.7s
⠦ 970a5a4847d9 Verifying Checksum 7.7s
⠦ 4f4fb700ef54 Download complete 7.7s
⠦ f1bbb1bc1e67 Waiting 7.7s
?25h?25l[+] Running 4/9
⠹ redis Pulling 13.2s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠧ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.8s
⠧ 970a5a4847d9 Verifying Checksum 7.8s
⠧ 4f4fb700ef54 Download complete 7.8s
⠧ f1bbb1bc1e67 Waiting 7.8s
?25h?25l[+] Running 4/9
⠸ redis Pulling 13.3s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠇ 00477ff4bbe5 Downloading 7.306MB/12.15M... 7.9s
⠇ 970a5a4847d9 Verifying Checksum 7.9s
⠇ 4f4fb700ef54 Download complete 7.9s
⠇ f1bbb1bc1e67 Waiting 7.9s
?25h?25l[+] Running 4/9
⠼ redis Pulling 13.4s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠏ 00477ff4bbe5 Downloading 7.306MB/12.15M... 8.0s
⠏ 970a5a4847d9 Verifying Checksum 8.0s
⠏ 4f4fb700ef54 Download complete 8.0s
⠏ f1bbb1bc1e67 Waiting 8.0s
?25h?25l[+] Running 4/9
⠴ redis Pulling 13.5s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠋ 00477ff4bbe5 Downloading 7.699MB/12.15M... 8.1s
⠋ 970a5a4847d9 Verifying Checksum 8.1s
⠋ 4f4fb700ef54 Download complete 8.1s
⠋ f1bbb1bc1e67 Waiting 8.1s
?25h?25l[+] Running 4/9
⠦ redis Pulling 13.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠙ 00477ff4bbe5 Downloading 10.06MB/12.15M... 8.2s
⠙ 970a5a4847d9 Verifying Checksum 8.2s
⠙ 4f4fb700ef54 Download complete 8.2s
⠙ f1bbb1bc1e67 Waiting 8.2s
?25h?25l[+] Running 4/9
⠧ redis Pulling 13.7s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠹ 00477ff4bbe5 Downloading 10.06MB/12.15M... 8.3s
⠹ 970a5a4847d9 Verifying Checksum 8.3s
⠹ 4f4fb700ef54 Download complete 8.3s
⠹ f1bbb1bc1e67 Waiting 8.3s
?25h?25l[+] Running 4/9
⠇ redis Pulling 13.8s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠸ 00477ff4bbe5 Downloading 11.88MB/12.15M... 8.4s
⠸ 970a5a4847d9 Verifying Checksum 8.4s
⠸ 4f4fb700ef54 Download complete 8.4s
⠸ f1bbb1bc1e67 Waiting 8.4s
?25h?25l[+] Running 4/9
⠏ redis Pulling 13.9s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠼ 00477ff4bbe5 Extracting 131.1kB/12.15... 8.5s
⠼ 970a5a4847d9 Verifying Checksum 8.5s
⠼ 4f4fb700ef54 Download complete 8.5s
⠼ f1bbb1bc1e67 Download complete 8.5s
?25h?25l[+] Running 4/9
⠋ redis Pulling 14.0s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠴ 00477ff4bbe5 Extracting 131.1kB/12.15... 8.6s
⠴ 970a5a4847d9 Verifying Checksum 8.6s
⠴ 4f4fb700ef54 Download complete 8.6s
⠴ f1bbb1bc1e67 Download complete 8.6s
?25h?25l[+] Running 4/9
⠙ redis Pulling 14.1s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠦ 00477ff4bbe5 Extracting 262.1kB/12.15... 8.7s
⠦ 970a5a4847d9 Verifying Checksum 8.7s
⠦ 4f4fb700ef54 Download complete 8.7s
⠦ f1bbb1bc1e67 Download complete 8.7s
?25h?25l[+] Running 4/9
⠹ redis Pulling 14.2s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠧ 00477ff4bbe5 Extracting 4.981MB/12.15... 8.8s
⠧ 970a5a4847d9 Verifying Checksum 8.8s
⠧ 4f4fb700ef54 Download complete 8.8s
⠧ f1bbb1bc1e67 Download complete 8.8s
?25h?25l[+] Running 4/9
⠸ redis Pulling 14.3s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠇ 00477ff4bbe5 Extracting 7.733MB/12.15... 8.9s
⠇ 970a5a4847d9 Verifying Checksum 8.9s
⠇ 4f4fb700ef54 Download complete 8.9s
⠇ f1bbb1bc1e67 Download complete 8.9s
?25h?25l[+] Running 5/9
⠼ redis Pulling 14.4s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠿ 00477ff4bbe5 Pull complete 8.9s
⠏ 970a5a4847d9 Extracting 100B/100B 9.0s
⠏ 4f4fb700ef54 Download complete 9.0s
⠏ f1bbb1bc1e67 Download complete 9.0s
?25h?25l[+] Running 6/9
⠴ redis Pulling 14.5s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠿ 00477ff4bbe5 Pull complete 8.9s
⠿ 970a5a4847d9 Pull complete 9.0s
⠋ 4f4fb700ef54 Extracting 32B/32B 9.1s
⠋ f1bbb1bc1e67 Download complete 9.1s
?25h?25l[+] Running 8/9
⠦ redis Pulling 14.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠿ 00477ff4bbe5 Pull complete 8.9s
⠿ 970a5a4847d9 Pull complete 9.0s
⠿ 4f4fb700ef54 Pull complete 9.1s
⠿ f1bbb1bc1e67 Pull complete 9.1s
?25h?25l[+] Running 9/9
⠿ redis Pulled 14.6s
⠿ c30352492317 Pull complete 3.7s
⠿ 2e707cb76875 Pull complete 3.7s
⠿ 41a20ff430bc Pull complete 4.0s
⠿ d326b14c5477 Pull complete 4.4s
⠿ 00477ff4bbe5 Pull complete 8.9s
⠿ 970a5a4847d9 Pull complete 9.0s
⠿ 4f4fb700ef54 Pull complete 9.1s
⠿ f1bbb1bc1e67 Pull complete 9.1s
?25h?25l[+] Building 0.0s (0/0)
?25h?25l[+] Building 0.0s (0/0)
?25h?25l[+] Building 0.0s (0/0)
?25h?25l[+] Building 0.1s (1/2)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
?25h?25l[+] Building 0.2s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.1s
?25h?25l[+] Building 0.4s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.2s
?25h?25l[+] Building 0.5s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.4s
?25h?25l[+] Building 0.7s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.5s
?25h?25l[+] Building 0.8s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.7s
?25h?25l[+] Building 1.0s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 0.8s
?25h?25l[+] Building 1.1s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.0s
?25h?25l[+] Building 1.3s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.1s
?25h?25l[+] Building 1.4s (2/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.3s
?25h?25l[+] Building 1.5s (3/3)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.4s
?25h?25l[+] Building 1.7s (8/9)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.4s
=> [1/5] FROM docker.io/library/python:3.10-slim@sha256:25f03d17398b3f00 0.0s
=> [internal] load build context 0.0s
=> => transferring context: 1.14kB 0.0s
=> CACHED [2/5] WORKDIR /code 0.0s
=> CACHED [3/5] COPY ./requirements.txt ./ 0.0s
=> CACHED [4/5] RUN pip install --no-cache-dir --upgrade -r requirements 0.0s
=> [5/5] COPY ./src ./src 0.1s
?25h?25l[+] Building 1.8s (9/9)
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.4s
=> [1/5] FROM docker.io/library/python:3.10-slim@sha256:25f03d17398b3f00 0.0s
=> [internal] load build context 0.0s
=> => transferring context: 1.14kB 0.0s
=> CACHED [2/5] WORKDIR /code 0.0s
=> CACHED [3/5] COPY ./requirements.txt ./ 0.0s
=> CACHED [4/5] RUN pip install --no-cache-dir --upgrade -r requirements 0.0s
=> [5/5] COPY ./src ./src 0.2s
?25h?25l[+] Building 1.8s (10/10) FINISHED
=> [internal] load build definition from Dockerfile 0.0s
=> => transferring dockerfile: 224B 0.0s
=> [internal] load .dockerignore 0.0s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:3.10-slim 1.4s
=> [1/5] FROM docker.io/library/python:3.10-slim@sha256:25f03d17398b3f00 0.0s
=> [internal] load build context 0.0s
=> => transferring context: 1.14kB 0.0s
=> CACHED [2/5] WORKDIR /code 0.0s
=> CACHED [3/5] COPY ./requirements.txt ./ 0.0s
=> CACHED [4/5] RUN pip install --no-cache-dir --upgrade -r requirements 0.0s
=> [5/5] COPY ./src ./src 0.2s
=> exporting to image 0.0s
=> => exporting layers 0.0s
=> => writing image sha256:b063f7a8ee0b5967254febde558c2bfade23600e4fc0e 0.0s
=> => naming to docker.io/library/compose-fastapi-server 0.0s
?25h?25l[+] Running 0/0
⠋ Network compose_default Creating 0.1s
?25h?25l[+] Running 1/1
⠿ Network compose_default Created 0.1s
⠋ Container compose-redis-1 Creating 0.1s
?25h?25l[+] Running 2/2
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Crea... 0.2s
⠋ Container compose-fastapi-server-1 Creating 0.0s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Crea... 0.2s
⠙ Container compose-fastapi-server-1 Creating 0.1s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.4s
⠿ Container compose-fastapi-server-1 Created 0.2s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.5s
⠿ Container compose-fastapi-server-1 Created 0.2s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.6s
⠿ Container compose-fastapi-server-1 Created 0.2s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.7s
⠿ Container compose-fastapi-server-1 Created 0.2s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.8s
⠿ Container compose-fastapi-server-1 Created 0.2s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Created 0.2s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Starting 0.8s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Starting 0.9s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Starting 1.0s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Starting 1.1s
?25h?25l[+] Running 2/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Starting 1.2s
?25h?25l[+] Running 3/3
⠿ Network compose_default Create... 0.1s
⠿ Container compose-redis-1 Star... 0.9s
⠿ Container compose-fastapi-server-1 Started 1.2s
?25h
Remark. Services which are still up will not be rebuilt. As such, these services can persist data and state while rebuilt services have reset state.
!docker compose ps
NAME IMAGE COMMAND SERVICE CREATED STATUS PORTS
compose-fastapi-server-1 compose-fastapi-server "uvicorn src.main:ap…" fastapi-server 1 second ago Up Less than a second 0.0.0.0:5678->5678/tcp, 0.0.0.0:8080->80/tcp
compose-redis-1 redis:alpine "docker-entrypoint.s…" redis 2 seconds ago Up Less than a second 6379/tcp
Making multiple requests:
!http :8080
HTTP/1.1 200 OK
content-length: 44
content-type: application/json
date: Wed, 27 Dec 2023 23:26:41 GMT
server: uvicorn
{
"message": "Hello world!",
"visit_count": "1"
}
!http :8080
HTTP/1.1 200 OK
content-length: 44
content-type: application/json
date: Wed, 27 Dec 2023 23:26:41 GMT
server: uvicorn
{
"message": "Hello world!",
"visit_count": "2"
}
Monitoring running services resource usage:
!docker stats --no-stream $(docker ps --format "{{.Names}}" | grep -w 'compose')
CONTAINER ID NAME CPU % MEM USAGE / LIMIT MEM % NET I/O BLOCK I/O PIDS
8176f2fae142 compose-fastapi-server-1 1.19% 54.91MiB / 3.841GiB 1.40% 3.5kB / 2.89kB 0B / 0B 4
5f79ae484a67 compose-redis-1 0.28% 2.82MiB / 3.841GiB 0.07% 2.92kB / 1.36kB 0B / 0B 5
Teardown:
!docker compose down
Show code cell output
?25l[+] Running 0/0
⠋ Container compose-fastapi-server-1 Stopping 0.1s
?25h?25l[+] Running 0/1
⠙ Container compose-fastapi-server-1 Stopping 0.2s
?25h?25l[+] Running 0/1
⠹ Container compose-fastapi-server-1 Stopping 0.3s
?25h?25l[+] Running 0/1
⠸ Container compose-fastapi-server-1 Stopping 0.4s
?25h?25l[+] Running 0/1
⠼ Container compose-fastapi-server-1 Stopping 0.5s
?25h?25l[+] Running 0/1
⠴ Container compose-fastapi-server-1 Stopping 0.6s
?25h?25l[+] Running 1/1
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠋ Container compose-redis-1 Stop... 0.0s
?25h?25l[+] Running 1/2
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠙ Container compose-redis-1 Stop... 0.1s
?25h?25l[+] Running 1/2
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠹ Container compose-redis-1 Stop... 0.2s
?25h?25l[+] Running 1/2
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠸ Container compose-redis-1 Stop... 0.3s
?25h?25l[+] Running 1/2
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠿ Container compose-redis-1 Remo... 0.5s
?25h?25l[+] Running 1/2
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠿ Container compose-redis-1 Remo... 0.5s
?25h?25l[+] Running 2/2
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠿ Container compose-redis-1 Remo... 0.6s
⠋ Network compose_default Removi... 0.1s
?25h?25l[+] Running 3/3
⠿ Container compose-fastapi-server-1 Removed 0.6s
⠿ Container compose-redis-1 Remo... 0.6s
⠿ Network compose_default Remove... 0.1s
?25h